Control Binding expressions
Building expressions to convert parameter values
In order to perform a Control conversion task, you must define the conversions or transformation of Parameter values from a source to a target. Where you are converting or importing data from an Instrument database or migrating from one control platform to another there will always be certain Parameter values that will require converting to fit the data to the target Control System platform.
-
For example a typical PID algorithm that resides in a Honeywell Basic Controller may use a Control Equation 'B' that when migrating to an HPM or Experion control system will require that Control Equation 'C' is selected to convert to the same control behavior. The Control Binding that is defined for this Parameter item will require that an expression is developed in C# code to support its value conversion.
-
When discussing Control binding a Property is the abstract of an algorithm or Function Block Parameter. Parameters defined in a Function Block are technically the properties for the block. The use of the word Parameter is a carry over from legacy Control System configuration.
-
A default Property Binding expression that is generated will simply take the value from the source of the Control Binding and provide it unchanged to the target specified in the Control Binding as shown below:
// Binded to Source Control Property Value [PVEUHI]
public System.Double Source_AIChannelA_PVEXEUHI;
// Converted Target Control Property
private System.Double _AIChannelA_PVEXEUHI;
[PropertyBinderAttribute("PVEUHI", "AIChannelA.PVEXEUHI")]
[PropertyRoundingAttribute("SubString(_PVFORMAT, 2, 1, 1)", true)]
[PropertyDefaultValueAttribute("102.9")]
public System.Double AIChannelA_PVEXEUHI
{
get
{
// TODO: modify this get() accessor method to accomplish
// Source Control Property value conversion
return Source_AIChannelA_PVEXEUHI;
}
set
{
_AIChannelA_PVEXEUHI = value;
}
}
-
Below is a code expression that provides modification to the value of the source Property prior to assigning it to the target Property of the Control Binding. This code expression is using the values of the source properties or parameters PVEUHI and PVEULO to calculate a default value to apply to the target Property PVEXLOLM.
-
| Note that the only valid place to modify a code expression for a Control Binding is in the get accessor of the Converted Target Control Property as indicated by the //TODO: comment.
|
// Binded to Source Control Property Value [PVEUHI]
public System.Double Source_AIChannelA_PVEXEUHI;
// Converted Target Control Property
private System.Double _AIChannelA_PVEXEUHI;
[PropertyBinderAttribute("PVEUHI", "AIChannelA.PVEXEUHI")]
[PropertyRoundingAttribute("SubString(_PVFORMAT, 2, 1, 1)", true)]
[PropertyDefaultValueAttribute("102.9")]
public System.Double AIChannelA_PVEXEUHI
{
get
{
// TODO: modify this get() accessor method to accomplish
// Source Control Property value conversion
// Notes: We use a string property to read the enumeration value of PVRNGOP.
// Note the use of variable names prefixed with the '_' character. You can use a SourceProperty
// reference by prefixing its name with the '_' character.
// Note that we use ToUpper() method to compare both values in upper case to eliminate having
// to worry about case sensitivity.
return _PVRNGOP.ToUpper() == "NONE"?
((_PVEUHI - _PVEULO) * 0.0689) + _PVEUHI
: ((_PVEUHI - _PVEULO) * 0.029) + _PVEUHI;
}
set
{
_AIChannelA_PVEXEUHI = value;
}
}
Constants
-
Constants can be specified as the source of Control bindings by simply selecting the <Constant> as the source Property. The value specified as its default will become the Constant value that is written to the Control Binding target Property during Control conversion execution. You can reference a custom variable as the source of the default value for a <Constant> type source Property.
-
User defined constants can be referenced in Control Binding code expressions such as:
return -(Source_DACA_PVEUHI - Source_DACA_PVEULO) * $<C_FF_PVEXEHI>$;
Constants used within script code must be enclosed within '$' characters.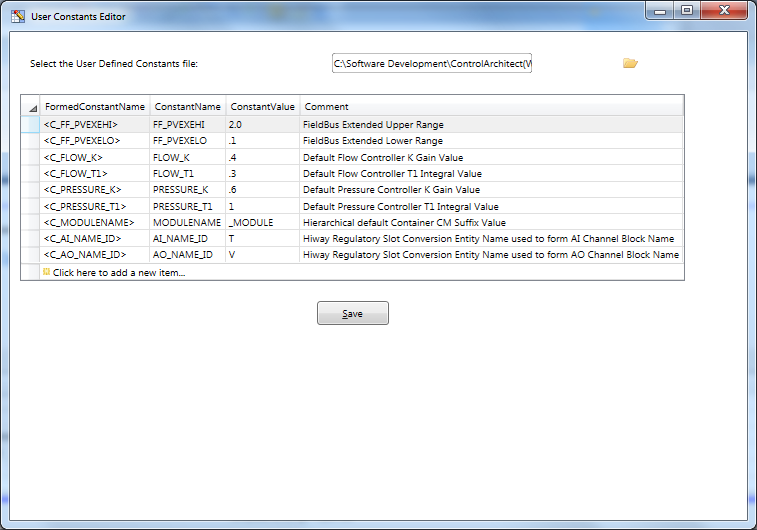
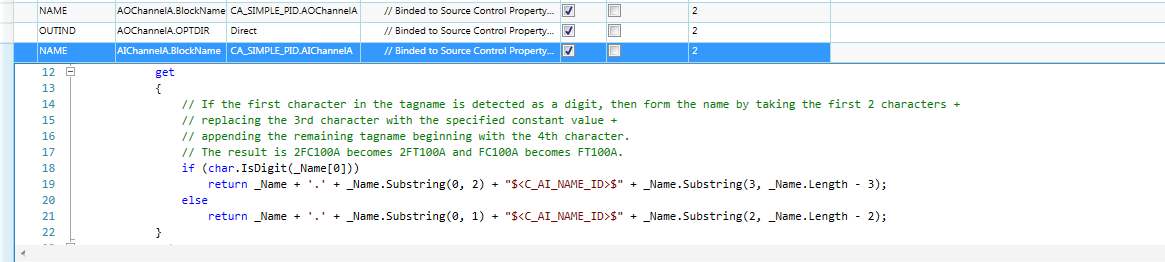
-
-
Users can develop a set of constants that are then referenced by the Control Binding templates. Using the Constants Editor in Control Architect constants can be defined that can then be referenced as source properties of Control bindings.
-
Constants when defined will be named using <C_UserName> where UserName specifies the unique identifier for this Constant. Typically a set of constants will be developed that defines the rules for a particular project or client site. Examples of common constants that may be defined include:
-
FFPVExtendedRangeUpperLimit - Foundation FieldBus type Process Measure Input Extended Upper Limit
-
FFPVExtendedRangeLowerLimit - Foundation FieldBus type Process Measure Input Extended Lower Limit
-
AnalogPVExtendedRangeUpperLimit - Standard 4-20 ma device type Process Measure Input Extended Upper Limit
-
AnalogPVExtendedRangeLowerLimit - Standard 4-20 ma device type Process Measure Input Extended Lower Limit
-
FlowPVLowCutOff - standard flow range percent Low Cutoff
-
K_FlowControllerGain - standard default Control Gain (K) value for flow service
-
T1_FlowControllerIntegral - standard default Control Integral (T1) value for flow service
-
K_PressureControllerGain - standard default Control Gain (K) value for pressure service
-
T1_PressureControllerIntegral - standard default Control Integral (T1) value for pressure service
Special Constants
-
Use <Constant> as the Source Property bound to <C_BASEDONFILE> as the Target Property in a Control Binding to overwrite the BasedOn Template filename that was used to define the set of Target Control properties. You would specify the pure Control Module name as the value without the file path and filename extension. The Control Module name specified as the value must reside in the folder path that is specified as your PKS Control Templates folder, which can be accessed from the Ribbon Toolbar File menu item.
Searching and Replacing Parameter Values
You can search and replace Parameter values by specifying the value of BLOCK.<PARAMETER> for the Target Control Property. You define this special Target Property in your Control Property Template as in the example below, which then allows you to select it for the Target Control Property in a Control Binding:
When searching and replacing Parameter values you will define a column in your Excel DataSource such as "TextSearch" that is used to specify the Parameter value to search for. The DefaultValueIfEmpty attribute for your Control Binding is used to specify the replacement value. When this type of Control Binding usage is detected during generation of a Data Collection task all Parameter values for all Control Modules and their embedded blocks will be searched for the value specified in the Excel Data Source.
You can specify optional attributes in the Parameter name format:
-
BLOCK.<PARAMETER-I> indicates to search for Parameter values while ignoring alpha case. This is the same as formatting the Property as BLOCK.<PARAMETER>.
-
BLOCK.<PARAMETER-C> indicates to search for Parameter values using alpha case sensitivity.
-
BLOCK.<PARAMETER-P> indicates to search for partial use of the specified Parameter value while ignoring alpha case. This would often be used to edit values specified in function descriptors, keywords, etc.
Excel DataSource:
Control Property Template:
Control Binding Template:
Searching and Replacing Parameter Connection References
You can search and replace Parameter Connection references by specifying the value of BLOCK.<CONNECTION> for the Target Control Property. You define this special Target Property in your Control Property Template as in the example above, which then allows you to select it for the Target Control Property in a Control Binding:
When searching and replacing Parameter Connection references you will define a column in your Excel DataSource such as "Find" that is used to specify the Parameter Connection reference to search for. The DefaultValueIfEmpty attribute for your Control Binding is used to specify the replacement Connection reference value. When this type of Control Binding usage is detected during generation of a Data Collection task all Control Module Parameter Connection references and expressions will be searched for the reference specified in the Control Binding Source property that defines the Excel Data Source column to use. In the example above the Control Binding source property is bound to the Excel Data Source column named Find. In the example above the replacement value is specified in the Control Binding DefaultValueIfEmpty attribute.
Parameter references are searched in the following elements:
-
Parameter reference connections
-
Expression type parameters such used in AuxCalc and RegCalc type Function blocks.
You can specify optional attributes in the Connection name format:
-
BLOCK.<CONNECTION> indicates to search for both input and output Parameter Connection references.
-
BLOCK.<CONNECTION-I> indicates to search for only input Parameter Connection references.
-
BLOCK.<CONNECTION-O> indicates to search for only output Parameter Connection references. This would be for Block types such as PUSH.
You can choose to find and replace using the wildcard character * in the format CMname.*:
-
Specify the find reference as in GEN_1.* and the replacement value as specified in DefaultValueIfEmpty GEN_1X. This will find all references to the Control Module "GEN_1" regardless of the BlockName or Parameter Name path. Any reference to the Control Module "GEN_1" will be replaced with "GEN_1X".
-
Parameter references such as "GEN_1.DACA.PV" would be replaced with "GEN_1X.DACA.PV"
-
Expression references such as "GEN_1.NUMERICA.PV > 10.0? 0.0 : GEN_1.NUMERICA.PV" would be replaced with "GEN_1X.NUMERICA.PV > 10.0? 0.0 : GEN_1X.NUMERICA.PV".
You can choose to find and replace multiple values by separating search and find values with the '|' pipe character:
-
Specify multiple find and replace values as in Find: "GEN_1.*|GEN_2.*" and Replace (DefaultValueIfEmpty): "GEN_1X|GEN_2X".
-
Use this format to replace multiple references in a Control Module. You cannot specify multiple Control Bindings using the Target Property name BLOCK.<CONNECTION>. The Control Binding view enforces uniqueness in the Target Property names that are specified in the Control Binding Template.
Block Functions
You can also execute custom functions such as deleting an embedded Function Block in a PKS Control Module. See Control Binding Functions.
Tips & Tricks
-
You can save script code as a Code Snippet to build a library of commonly used expressions.
-
You can use the key combination Ctrl+k, followed by the 'F' key to format a code snippet.
-
You can restore the Default Value based on the value found in the BasedOn Template by pressing the F9 key for a selected Control Binding item.
-
You can modify a Control Binding script so that it ignores empty values in an Excel DataSource. Modify a Control Binding script such that the result value is its Target Control Property if the Excel Source Property value is empty.
// Binded to Source Control Property Value [DACA_EUDESC]
public System.String Source_DACA_EUDESC;
// Converted Target Control Property
private System.String _DACA_EUDESC;
[PropertyBinderAttribute("DACA.EUDESC", "DACA.EUDESC")]
[PropertyRoundingAttribute("2", false)]
[PropertyDefaultValueAttribute("")]
public System.String DACA_EUDESC
{
get
{
// If the EUDESC value in the Excel DataSource is empty, then just return
// the existing Entity Parameter value, which will result in no detection
// of changes.
if (string.IsNullOrWhiteSpace(Source_DACA_EUDESC)) return _DACA_EUDESC;
return Source_DACA_EUDESC;
}
set
{
_DACA_EUDESC = value;
}
}